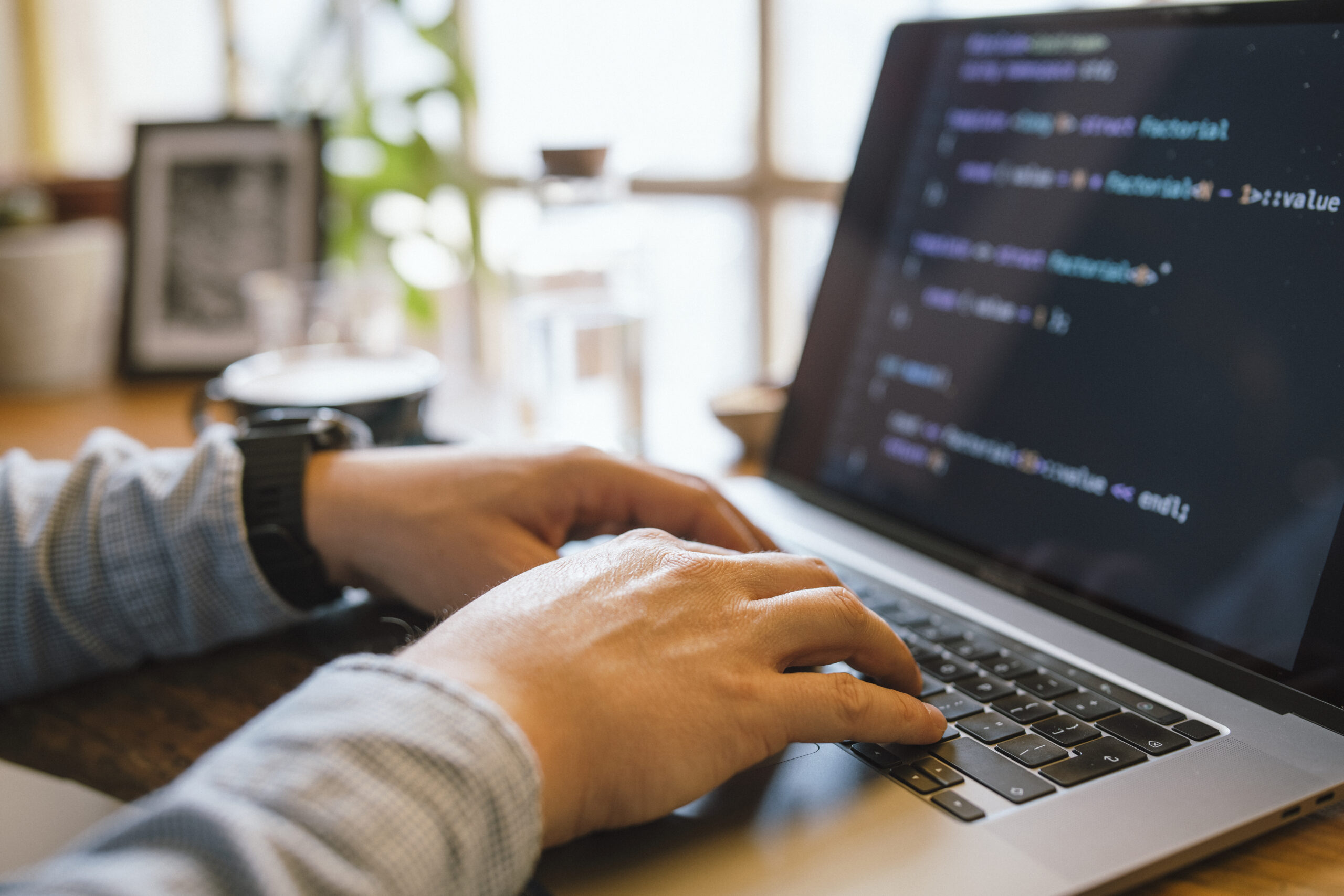
Debugging is One of the more essential — but typically missed — skills inside a developer’s toolkit. It isn't pretty much correcting damaged code; it’s about understanding how and why things go Incorrect, and Understanding to Assume methodically to unravel challenges competently. Irrespective of whether you are a starter or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and dramatically improve your efficiency. Listed below are a number of methods to assist builders stage up their debugging match by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the applications they use on a daily basis. Even though composing code is 1 part of progress, recognizing tips on how to communicate with it effectively all through execution is Similarly essential. Fashionable development environments appear equipped with impressive debugging abilities — but numerous developers only scratch the surface area of what these tools can perform.
Consider, such as, an Integrated Development Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, step by code line by line, and in many cases modify code within the fly. When made use of appropriately, they Permit you to observe accurately how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-conclusion builders. They allow you to inspect the DOM, keep an eye on community requests, check out serious-time efficiency metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can switch disheartening UI concerns into workable tasks.
For backend or process-level developers, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than functioning processes and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging efficiency issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Handle programs like Git to comprehend code historical past, obtain the precise moment bugs had been introduced, and isolate problematic adjustments.
Ultimately, mastering your tools indicates heading over and above default options and shortcuts — it’s about producing an personal knowledge of your improvement atmosphere in order that when troubles come up, you’re not dropped at nighttime. The greater you are aware of your applications, the greater time you may shell out fixing the particular challenge as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before leaping to the code or building guesses, builders want to create a dependable natural environment or circumstance exactly where the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, generally resulting in wasted time and fragile code changes.
The initial step in reproducing an issue is accumulating just as much context as you possibly can. Talk to inquiries like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it turns into to isolate the precise problems under which the bug happens.
After you’ve gathered adequate information, try and recreate the problem in your neighborhood environment. This may suggest inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue seems intermittently, think about producing automatic exams that replicate the sting conditions or state transitions included. These tests not merely assistance expose the trouble and also prevent regressions Later on.
From time to time, The problem can be environment-certain — it would materialize only on particular working devices, browsers, or below distinct configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mentality. It requires patience, observation, as well as a methodical strategy. But once you can regularly recreate the bug, you are presently halfway to repairing it. Using a reproducible circumstance, You may use your debugging tools more effectively, test possible fixes safely, and communicate more Evidently with all your workforce or buyers. It turns an summary grievance right into a concrete problem — and that’s the place developers thrive.
Read and Understand the Mistake Messages
Mistake messages in many cases are the most useful clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to take care of mistake messages as direct communications in the system. They normally inform you just what happened, where by it transpired, and often even why it occurred — if you know how to interpret them.
Start out by looking through the message diligently and in entire. Numerous builders, particularly when under time force, glance at the main line and promptly start out producing assumptions. But further while in the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste error messages into search engines like google and yahoo — read through and understand them initially.
Break the mistake down into parts. Could it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate brought on it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some mistakes are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective instruments inside of a developer’s debugging toolkit. When made use of effectively, it offers real-time insights into how an software behaves, encouraging you understand what’s going on under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with recognizing what to log and at what amount. Prevalent logging levels include DEBUG, Facts, Alert, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic info during development, INFO for general situations (like prosperous start off-ups), WARN for potential issues that don’t crack the appliance, ERROR for actual problems, and Lethal once the method can’t go on.
Prevent read more flooding your logs with extreme or irrelevant information. An excessive amount of logging can obscure important messages and decelerate your program. Focus on critical functions, state improvements, input/output values, and demanding decision factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially precious in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-considered-out logging approach, it is possible to reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and dependability within your code.
Feel Just like a Detective
Debugging is not merely a technical job—it's a sort of investigation. To correctly determine and resolve bugs, builders must method the method just like a detective fixing a thriller. This mentality helps break down complicated concerns into workable pieces and follow clues logically to uncover the root cause.
Begin by gathering evidence. Look at the signs of the trouble: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect just as much applicable information as you can without jumping to conclusions. Use logs, check circumstances, and user reports to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Check with on your own: What may very well be triggering this conduct? Have any modifications lately been made into the codebase? Has this difficulty transpired just before beneath equivalent situations? The goal should be to slim down prospects and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the challenge inside a managed setting. Should you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code issues and Allow the effects direct you closer to the reality.
Pay shut interest to compact information. Bugs often hide from the least predicted locations—similar to a missing semicolon, an off-by-just one error, or maybe a race situation. Be extensive and client, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface later on.
Finally, continue to keep notes on Everything you tried out and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in advanced techniques.
Produce Checks
Writing tests is one of the best solutions to help your debugging skills and All round growth performance. Tests not just aid catch bugs early and also function a security Internet that provides you self esteem when earning changes to your codebase. A properly-examined software is simpler to debug as it means that you can pinpoint particularly wherever and when a dilemma takes place.
Get started with device assessments, which deal with unique capabilities or modules. These smaller, isolated assessments can promptly reveal no matter whether a particular piece of logic is Operating as expected. When a check fails, you instantly know exactly where to look, noticeably lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—troubles that reappear right after Earlier getting fixed.
Future, combine integration exams and end-to-conclusion assessments into your workflow. These assist ensure that several areas of your application function alongside one another efficiently. They’re especially practical for catching bugs that arise in complicated systems with many components or products and services interacting. If anything breaks, your tests can tell you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect effectively, you need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust initial step. As soon as the check fails continually, you are able to target correcting the bug and view your examination go when the issue is settled. This technique makes certain that exactly the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable course of action—encouraging you catch much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a difficult situation, it’s quick to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping absent. Getting breaks can help you reset your intellect, cut down irritation, and infrequently see The difficulty from the new point of view.
If you're far too near to the code for way too prolonged, cognitive tiredness sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be less efficient at trouble-resolving. A brief stroll, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specifically throughout longer debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, but it surely truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature being a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural concern, each can train you a little something beneficial should you make time to replicate and review what went wrong.
Begin by asking oneself a number of key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and allow you to Create much better coding behaviors transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent faults—you could proactively prevent.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially highly effective. No matter whether it’s by way of a Slack information, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the similar concern boosts team effectiveness and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the most effective developers are usually not the ones who write best code, but those that repeatedly discover from their problems.
In the end, Every single bug you fix adds a completely new layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to become superior at what you do.